Flag <<
Previous Next >> GD 圖形庫中的函數
ROC單一菱形
#include <stdio.h>
#include <gd.h>
#include <math.h>
void draw_roc_flag(gdImagePtr img);
void draw_single_diamond(gdImagePtr img, int center_x, int center_y, int sun_radius, int color);
int main() {
// width 3: height 2
int width = 1200;
// 國旗長寬比為 3:2
int height = (int)(width * 2.0 / 3.0);
gdImagePtr img = gdImageCreateTrueColor(width, height);
gdImageAlphaBlending(img, 0);
draw_roc_flag(img);
FILE *outputFile = fopen("./../images/single_diamond.png", "wb");
if (outputFile == NULL) {
fprintf(stderr, "Error opening the output file.\n");
return 1;
}
gdImagePngEx(img, outputFile, 9);
fclose(outputFile);
gdImageDestroy(img);
return 0;
}
void draw_roc_flag(gdImagePtr img) {
int width = gdImageSX(img);
int height = gdImageSY(img);
int red, white, blue;
int center_x = (int)(width / 4);
int center_y = (int)(height / 4);
int sun_radius = (int)(width / 8);
red = gdImageColorAllocate(img, 255, 0, 0); // 紅色
white = gdImageColorAllocate(img, 255, 255, 255); // 白色
blue = gdImageColorAllocate(img, 0, 0, 149); // 藍色
gdImageFilledRectangle(img, 0, 0, width, height, red);
gdImageFilledRectangle(img, 0, 0, (int)(width / 2.0), (int)(height / 2.0), blue);
draw_single_diamond(img, center_x, center_y, sun_radius, white);
}
void draw_single_diamond(gdImagePtr img, int center_x, int center_y, int sun_radius, int color) {
float deg = M_PI / 180;
float sr = sun_radius / tan(75 * deg);
int ax, ay, bx, by, dx, dy, ex, ey;
gdPoint points[4];
ax = center_x;
ay = center_y - sun_radius;
bx = center_x - sun_radius * tan(15 * deg);
by = center_y;
ex = center_x;
ey = center_y + sun_radius;
dx = center_x + sun_radius * tan(15 * deg);
dy = center_y;
// A
points[0].x = ax + sun_radius * sin(30 * deg);
points[0].y = ay + sun_radius - sun_radius * cos(30 * deg);
// B
points[1].x = bx + sr - sr * cos(30 * deg);
points[1].y = by - sr * sin(30 * deg);
// E
points[2].x = ex - sun_radius * sin(30 * deg);
points[2].y = ey - (sun_radius - sun_radius * cos(30 * deg));
// D
points[3].x = dx - (sr - sr * cos(30 * deg));
points[3].y = dy + sr * sin(30 * deg);
// 對單一菱形區域範圍塗色
gdImageFilledPolygon(img, points, 4, color);
// 在菱形區域外圍畫線, 明確界定菱形範圍
gdImagePolygon(img, points, 4, color);
}
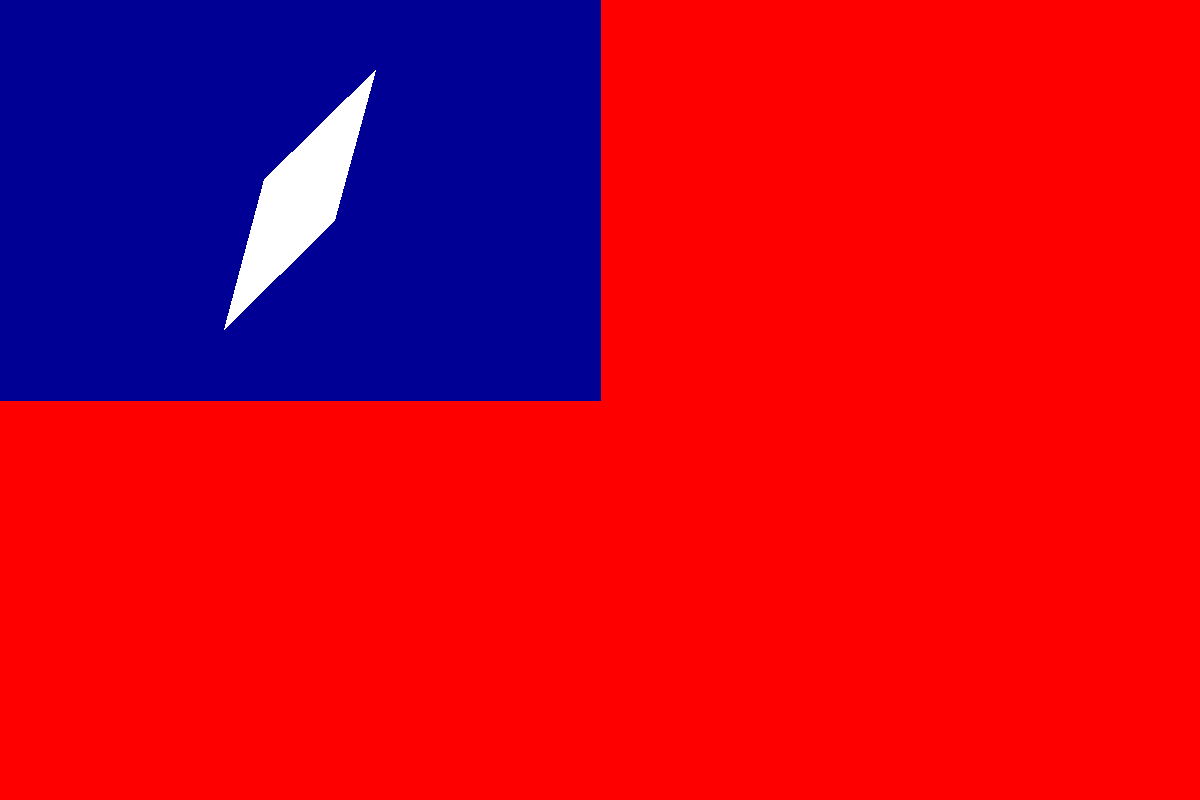
這個函數首先計算菱形的各個點的坐標(A、B、E、D),然後使用這些坐標繪製一個填充的菱形。最後,使用 gdImageFilledPolygon 函數填充菱形區域,同時使用 gdImagePolygon 函數在菱形外圍畫線,以明確界定菱形的範圍。
ax = center_x;
ay = center_y - sun_radius;
bx = center_x - sun_radius * tan(15 * deg);
by = center_y;
ex = center_x;
ey = center_y + sun_radius;
dx = center_x + sun_radius * tan(15 * deg);
dy = center_y;
ax 和 ay:菱形的頂點 A 的坐標,其 x 坐標等於中心點的 center_x,y 坐標等於中心點 center_y 減去太陽半徑 sun_radius。
bx 和 by:菱形的頂點 B 的坐標,其 x 坐標等於中心點的 center_x 減去太陽半徑 sun_radius 與角度 15 度的正切乘積,y 坐標等於中心點 center_y。
ex 和 ey:菱形的頂點 E 的坐標,其 x 坐標等於中心點的 center_x,y 坐標等於中心點 center_y 加上太陽半徑 sun_radius。
dx 和 dy:菱形的頂點 D 的坐標,其 x 坐標等於中心點的 center_x 加上太陽半徑 sun_radius 與角度 15 度的正切乘積,y 坐標等於中心點 center_y。
這樣計算出的坐標可以用於繪製一個正菱形。
// A
points[0].x = ax + sun_radius * sin(30 * deg);
points[0].y = ay + sun_radius - sun_radius * cos(30 * deg);
// B
points[1].x = bx + sr - sr * cos(30 * deg);
points[1].y = by - sr * sin(30 * deg);
// E
points[2].x = ex - sun_radius * sin(30 * deg);
points[2].y = ey - (sun_radius - sun_radius * cos(30 * deg));
// D
points[3].x = dx - (sr - sr * cos(30 * deg));
points[3].y = dy + sr * sin(30 * deg);
points[0] 對應頂點 A,其 x 坐標等於 ax 加上 sun_radius 乘以角度為 30 度的正弦值,y 坐標等於 ay 加上 sun_radius 減去 sun_radius 乘以角度為 30 度的餘弦值。
points[1] 對應頂點 B,其 x 坐標等於 bx 加上 sr 減去 sr 乘以角度為 30 度的餘弦值,y 坐標等於 by 減去 sr 乘以角度為 30 度的正弦值。
points[2] 對應頂點 E,其 x 坐標等於 ex 減去 sun_radius 乘以角度為 30 度的正弦值,y 坐標等於 ey 減去 sun_radius 減去 sun_radius 乘以角度為 30 度的餘弦值。
points[3] 對應頂點 D,其 x 坐標等於 dx 減去 sr 減去 sr 乘以角度為 30 度的餘弦值,y 坐標等於 dy 加上 sr 乘以角度為 30 度的正弦值。
這樣就確定了繪製一個正菱形所需的四個頂點的坐標。
for (int i=1;i<=6;i++){
這個 for 循環用於執行以下的繪製步驟,循環 6 次。每次循環,都會繪製一個單獨的菱形。就可以完成太陽
Flag <<
Previous Next >> GD 圖形庫中的函數